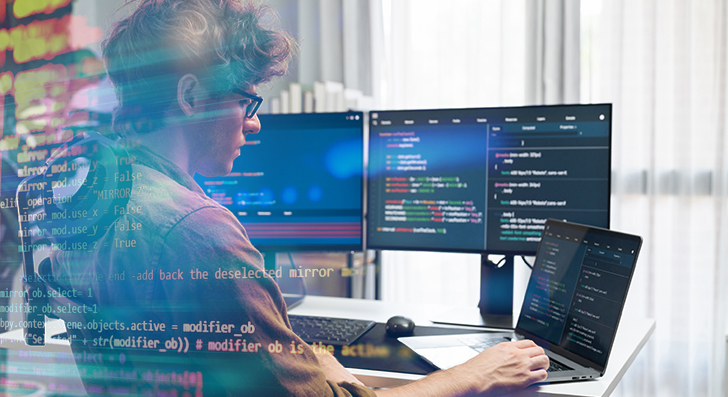
Scalability means your application can deal with growth—extra people, a lot more information, and much more traffic—without the need of breaking. Being a developer, developing with scalability in your mind saves time and stress later on. Right here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be portion of your system from the beginning. A lot of applications fall short once they improve quick for the reason that the initial structure can’t manage the additional load. Like a developer, you need to Consider early about how your process will behave under pressure.
Start off by creating your architecture being flexible. Stay clear of monolithic codebases wherever every little thing is tightly related. As an alternative, use modular design or microservices. These styles break your application into scaled-down, independent sections. Every module or provider can scale on its own without the need of affecting The full system.
Also, think of your databases from day one particular. Will it need to deal with one million customers or merely 100? Choose the appropriate type—relational or NoSQL—determined by how your details will increase. Approach for sharding, indexing, and backups early, even if you don’t need to have them still.
Another essential position is to stop hardcoding assumptions. Don’t generate code that only functions below existing ailments. Think about what would take place If the person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use structure patterns that assistance scaling, like message queues or occasion-driven techniques. These assist your app take care of far more requests devoid of having overloaded.
When you build with scalability in your mind, you are not just making ready for fulfillment—you might be lessening future headaches. A perfectly-prepared procedure is less complicated to take care of, adapt, and mature. It’s superior to get ready early than to rebuild later on.
Use the proper Database
Deciding on the ideal databases can be a crucial Portion of developing scalable applications. Not all databases are crafted the exact same, and using the Incorrect one can gradual you down as well as result in failures as your app grows.
Get started by comprehending your data. Can it be remarkably structured, like rows within a desk? If Of course, a relational database like PostgreSQL or MySQL is a good healthy. These are typically sturdy with associations, transactions, and regularity. They also aid scaling tactics like study replicas, indexing, and partitioning to take care of far more site visitors and data.
In the event your knowledge is more adaptable—like user action logs, product catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured details and can scale horizontally additional easily.
Also, take into account your browse and compose styles. Do you think you're doing numerous reads with fewer writes? Use caching and browse replicas. Are you presently handling a weighty generate load? Consider databases that could tackle higher publish throughput, or even occasion-dependent information storage devices like Apache Kafka (for temporary info streams).
It’s also clever to think forward. You may not have to have advanced scaling functions now, but picking a databases that supports them suggests you received’t have to have to modify afterwards.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your facts based upon your access patterns. And usually check database functionality when you mature.
In short, the proper database depends upon your app’s structure, speed desires, And just how you be expecting it to improve. Choose time to select correctly—it’ll preserve a great deal of difficulties later on.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, just about every modest delay adds up. Poorly penned code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s vital that you Develop effective logic from the start.
Get started by crafting clear, straightforward code. Steer clear of repeating logic and take away just about anything unwanted. Don’t select the most complex Alternative if an easy a single functions. Keep the features short, centered, and simple to check. Use profiling instruments to discover bottlenecks—places where by your code normally takes way too lengthy to operate or makes use of too much memory.
Upcoming, examine your databases queries. These normally sluggish matters down a lot more than the code alone. Be sure each query only asks for the info you really have to have. Stay away from SELECT *, which fetches almost everything, and rather decide on specific fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Particularly throughout large tables.
In case you notice the identical details getting asked for again and again, use caching. Retailer the effects temporarily making use of applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your database operations any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and would make your application more effective.
Make sure to check with huge datasets. Code and queries that get the job done great with 100 records may well crash if they have to take care of one million.
In short, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These techniques assistance your software continue to be sleek and responsive, at the same time as the load increases.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of more users and much more site visitors. If every little thing goes by way of one particular server, it is going to promptly turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these equipment aid maintain your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout many servers. In place of just one server undertaking every one of the operate, the load balancer routes end users to diverse servers determined by availability. This implies no single server receives overloaded. If a person server goes down, the load balancer can send visitors to the Other folks. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing info temporarily so it might be reused speedily. When customers ask for precisely the same details again—like an item webpage or perhaps a profile—you don’t really need to fetch it with the database when. You may serve it within the cache.
There are 2 common sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapidly access.
two. Client-facet caching (like browser caching or CDN caching) shops static data files close to the consumer.
Caching lowers database load, enhances velocity, and helps make your application a lot more economical.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when data does adjust.
In short, load balancing and caching are basic but impressive resources. Jointly, they help your app cope with more buyers, remain rapidly, and Get better from issues. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you require applications that let your app expand quickly. That’s where cloud platforms and containers come in. They provide you versatility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you'll need them. You don’t must get components or guess long run ability. When targeted visitors raises, you'll be able to incorporate far more methods with just a couple clicks or mechanically applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also give products and services like managed databases, storage, load balancing, and stability applications. You may deal with setting up your application in place of taking care of infrastructure.
Containers are One more crucial Instrument. A container packages your application and all the things it ought to operate—code, libraries, settings—into 1 unit. This can make it uncomplicated to move your app between environments, from a notebook to your cloud, devoid of surprises. Docker is the most well-liked Instrument for this.
Once your application employs numerous containers, tools like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to individual elements of your application into providers. You can update or scale pieces independently, that's great for effectiveness and dependability.
To put it briefly, employing cloud and container resources suggests you'll be able to scale speedy, deploy very easily, and Get better speedily when problems come about. If you want your application to grow with no limits, commence applying these equipment early. They help you save time, decrease possibility, and assist you to keep centered on developing, not repairing.
Observe Every little thing
For those who don’t keep track of your application, you received’t know when things go Improper. Checking allows you see how your app is undertaking, location problems early, and make greater conclusions as your application grows. It’s a important Portion of making scalable systems.
Begin by tracking standard metrics like CPU use, memory, disk House, and response time. These tell you how your servers and providers are undertaking. Applications like website Prometheus, Grafana, Datadog, or New Relic can help you acquire and visualize this knowledge.
Don’t just watch your servers—monitor your app as well. Keep watch over just how long it requires for end users to load web pages, how frequently glitches materialize, and where by they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s going on within your code.
Build alerts for significant challenges. Such as, In the event your reaction time goes earlier mentioned a Restrict or possibly a support goes down, you ought to get notified right away. This aids you resolve problems quick, often before buyers even detect.
Checking is additionally helpful when you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again prior to it causes authentic hurt.
As your app grows, targeted visitors and facts boost. Without checking, you’ll skip indications of difficulties until finally it’s too late. But with the appropriate resources set up, you remain in control.
Briefly, monitoring can help you keep your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about being familiar with your technique and making sure it really works well, even stressed.
Final Feelings
Scalability isn’t only for huge companies. Even modest applications want a solid foundation. By coming up with cautiously, optimizing correctly, and using the proper applications, you'll be able to Establish apps that improve smoothly with no breaking stressed. Begin modest, think huge, and Develop clever.